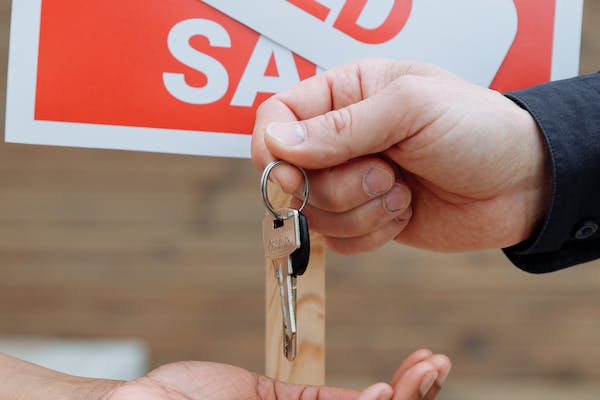
Introduction
In delegation, you delegate a certain request to an object to a second object, which we call the delegate. This is almost like a subclass sending a request to its parent class. In Rust we can achieve this by embedding the delegate, that is the object to which the original request is delegated, in the struct.
A best practice is, is that both the enclosing struct and the delegate implement the same trait.
Implementation in Rust
In this example we will implement a virtual UI with a TextWindow which has a width and a height, both are integer. For our UI it is important to know that area of the window.
We will start by implementing the Bounds trait which has one method:
trait Bounds {
fn area(&self) -> i32;
}
Note that because this method does not change the state of the implementing struct, we do not need &mut self.
Next we implement the Rectange struct:
#[derive(Clone)]
struct Rectangle {
width: i32,
height: i32,
}
impl Rectangle {
fn new(width: i32,height: i32)->Self {
Rectangle {
width,
height,
}
}
}
impl Bounds for Rectangle {
fn area(&self) -> i32 {
self.width*self.height
}
}
Some remarks:
- A rectangle in our example just has an integer width and an integer height
- We also implement a simple constructor-like method
- Note that we need to make sure that this struct is cloneable. This is needed for the embedding in the TextWindow struct later on
- Implementing the Bounds interface consisted of implementing one method without any side-effects.
Then we come to already mentioned TextWindow struct:
struct TextWindow {
title: String,
window: Rectangle
}
impl TextWindow {
fn new(title: &str,rectangle: &Rectangle) -> Self {
TextWindow {
title: title.to_string(),
window: rectangle.clone()
}
}
}
impl Bounds for TextWindow {
fn area(&self) -> i32 {
self.window.area()
}
}
Line by line:
- A textwindow in our example consists of a title string, and a rectangle called window.
- Like in Rectangle we also implement a constructor-like method
- Note that we clone the supplied Rectangle struct, because TextWindow talkes ownership of the supplied struct. If you want to avoid cloning, you would need to change the ownership structure of your
TextWindow
to store a reference to theRectangle
, but that would introduce lifetime constraints, and you wouldn’t be able to implement theBounds
trait as easily. Cloning is a valid approach in this case to simplify ownership. - In the implementation of the Bounds trait, the call to area() is delegated to an instance of the Rectangle struct. This is where the delegation happens.
Time to test
Now we can test our setup:
fn main() {
let rectangle=Rectangle::new(80,40);
let window=TextWindow::new("my window",&rectangle);
println!("The area is {} for window with title {}", window.area(),window.title);
}
Line by line:
- We create a new Rectangle struct
- We pass that along with a title to the constructor of the TextWindow
- Then we simply print out the title and the area.
Conclusion
Implementing this pattern was relatively easy. In a later post I will probably develop a more involved example, where the power of this relatively simple pattern becomes even clearer.