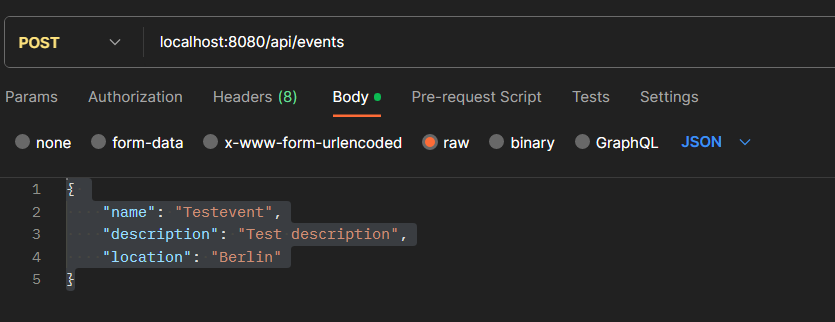
How to build a REST Api with Rust, Diesel and Postgres, part 2: Kubernetes
Introduction In this post I described how to build a simple Web API in Rust with Actix, Diesel,...
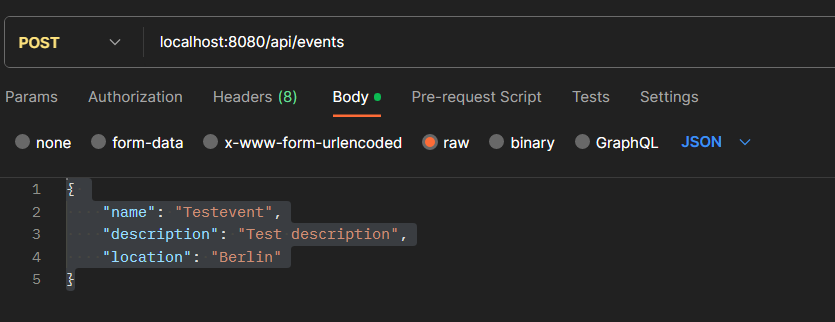
How to build a REST Api with Rust, Diesel and Postgres, part 1: setting up
Introduction For the past year I have been experimenting with Rust. So, now it is time for a...
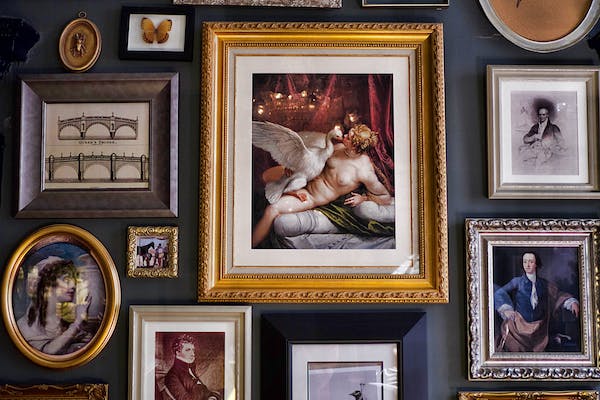
The Decorator pattern: an easy way to add functionality
Introduction The Decorator pattern can be used to dynamically alter or add functionality to existing classes. This pattern...
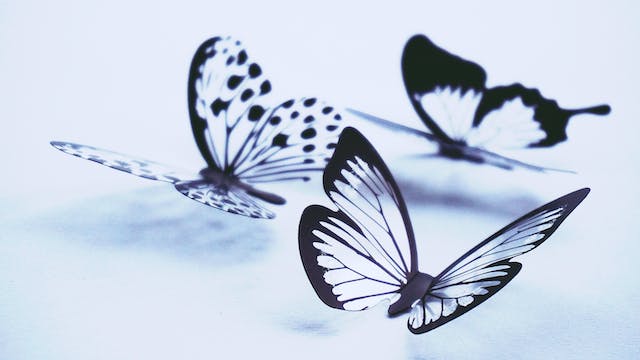
Design Patterns in Rust: Flyweight or go easy on your memory
Introduction The flyweight pattern is a pattern that helps minimize memory usage by sharing and reusing data. A...
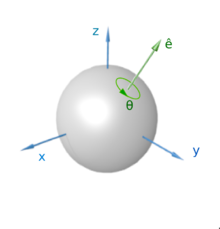
A simple quaternion library or a lesson in Operator Overloading
Introduction Just for fun and to try out Rust operator overloading capabilities, I decided to write a quaternion...
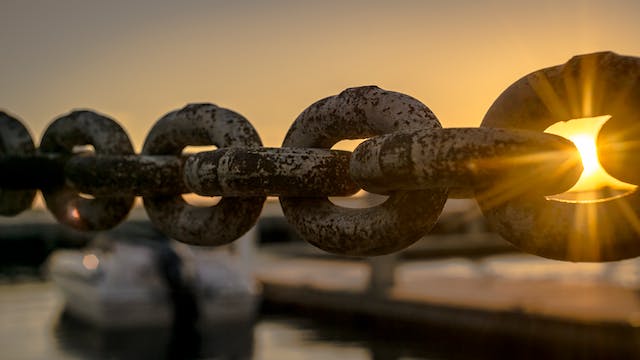
Design Patterns in Rust: Chain of Responsibility: there is more than one way to do it
Introduction The Chain of Responsibility (CoC) pattern describes a chain of command/request receivers. The client has no idea...
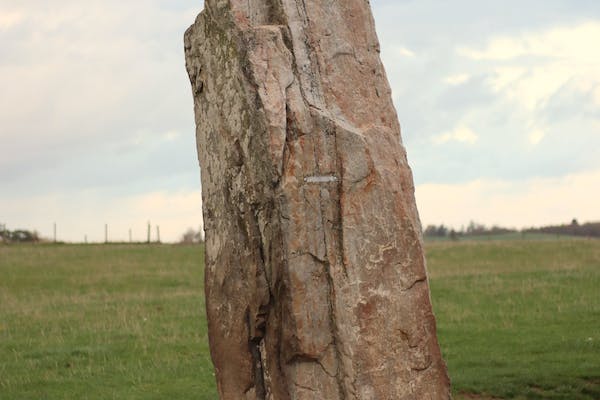
Design Patterns in Rust: Singleton, a unique way of creating objects in a threadsafe way
Introduction The singleton pattern restricts the instantiation of a class to a single instance. The singleton pattern makes...
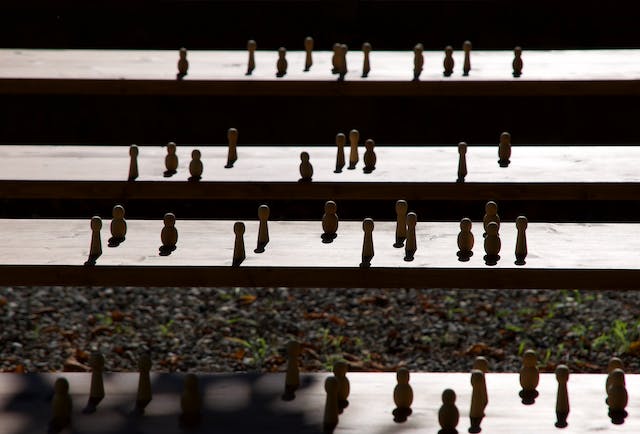
Design Patterns in Rust: The Command, a simple implementation of a versatile pattern
Introduction The command pattern is a behavioral design pattern. It is used by an Invoker to perform one action or...
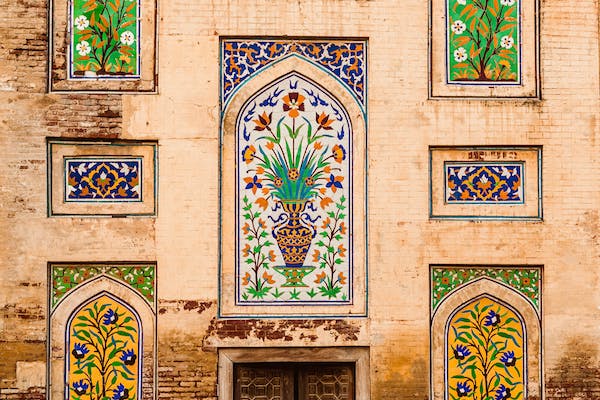
Design Patterns in Rust: Facade, hiding a complex world
Introduction The facade pattern is used as a way to hide more complex logic. A facade can do...
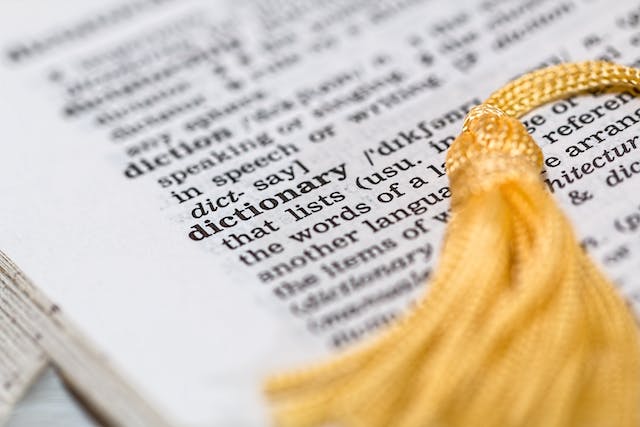
Design Patterns in Rust: Interpreter, making sense of the world
Introduction The Interpreter pattern can be used to interpret and evaluate sentences in a language. The idea is...