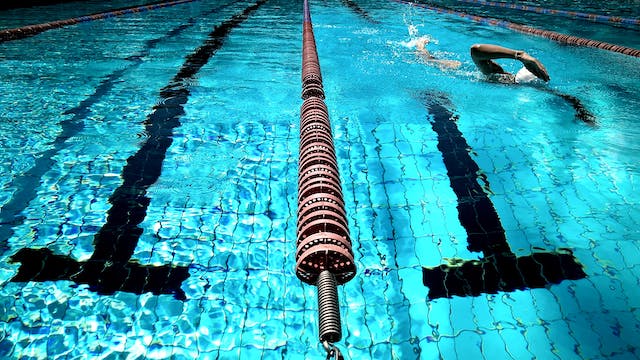
Easy Object Pool Management in Rust: Automating Release for Efficiency
Introduction In a previous article, we discussed the implementation of a thread-pool. One problem with this implementation is...
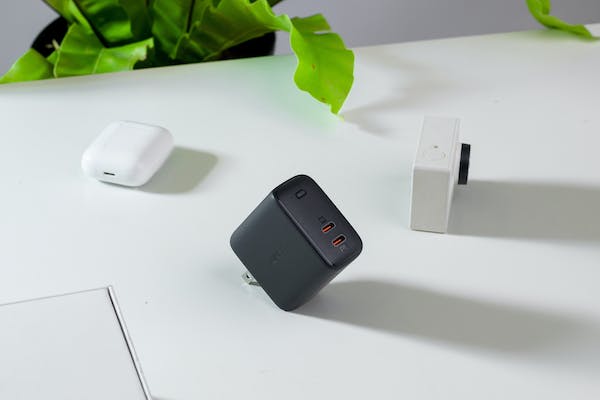
Easy Patterns in Rust: The Adapter Pattern
Introduction The Adapter pattern is used to make one interface compatible with another. It allows objects with different,...
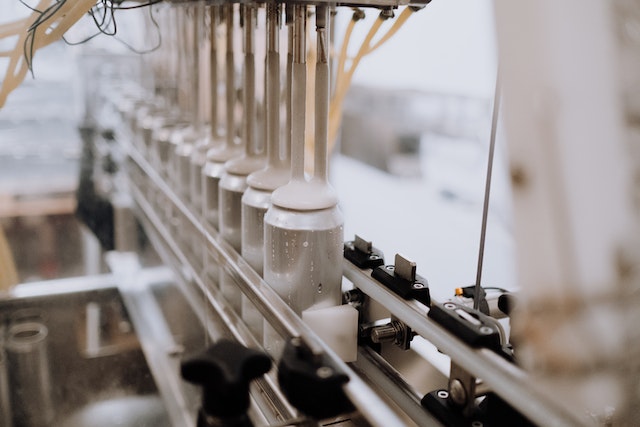
Design Patterns in Rust: Factory method, automating the factory
Introduction In this article I discussed the implementation of the Abstract Factory pattern. The Factory Method is simply an extension...
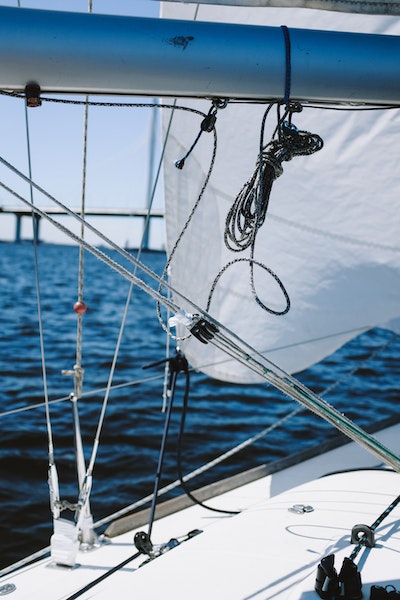
An easy way of implementing the Dependency Injection Pattern in Rust
Introduction Dependency Injection is simply said, the idea that your classes should depend on abstraction, i.e. the abstraction...
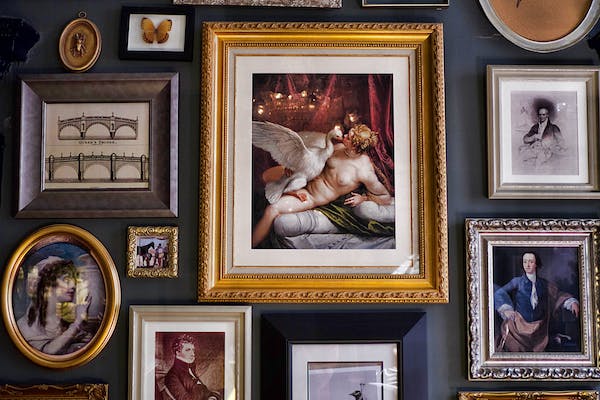
The Decorator pattern: an easy way to add functionality
Introduction The Decorator pattern can be used to dynamically alter or add functionality to existing classes. This pattern...
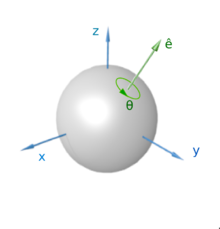
A simple quaternion library or a lesson in Operator Overloading
Introduction Just for fun and to try out Rust operator overloading capabilities, I decided to write a quaternion...
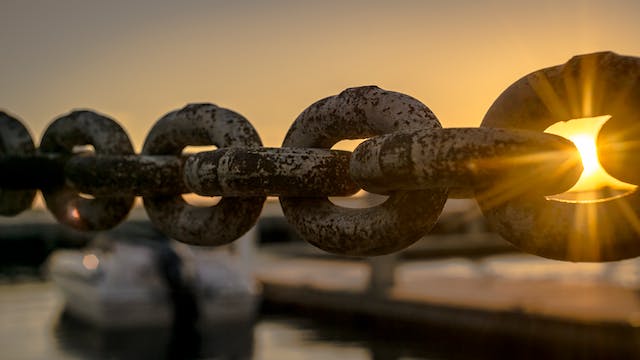
Design Patterns in Rust: Chain of Responsibility: there is more than one way to do it
Introduction The Chain of Responsibility (CoC) pattern describes a chain of command/request receivers. The client has no idea...
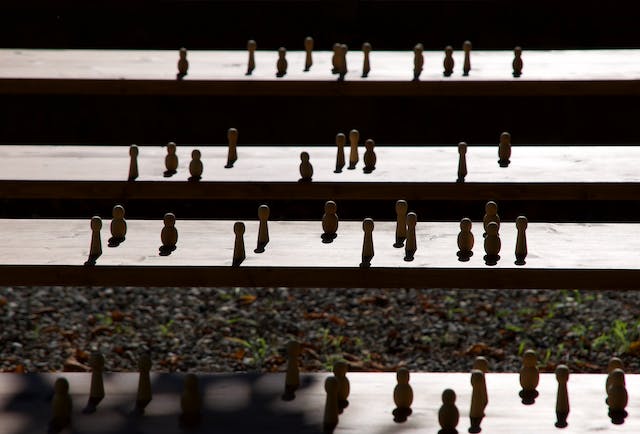
Design Patterns in Rust: The Command, a simple implementation of a versatile pattern
Introduction The command pattern is a behavioral design pattern. It is used by an Invoker to perform one action or...
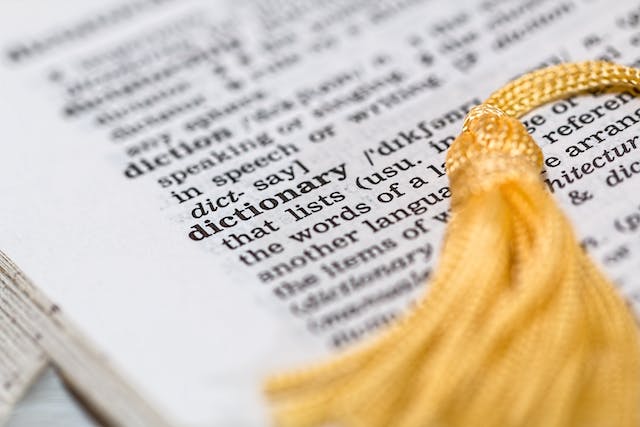
Design Patterns in Rust: Interpreter, making sense of the world
Introduction The Interpreter pattern can be used to interpret and evaluate sentences in a language. The idea is...
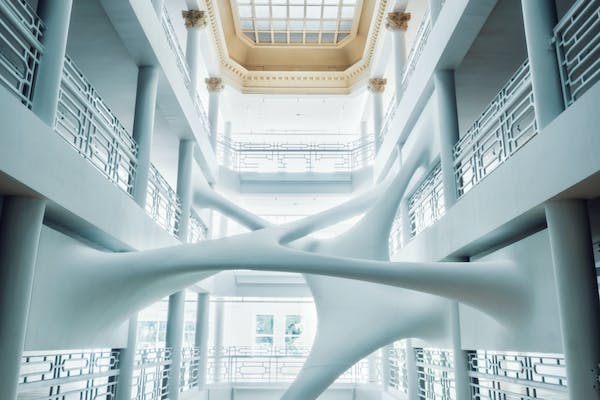
Design Patterns in Rust: Mediator, or uncoupling objects made easy
Introduction The mediator pattern is a pettern used when you want to simplify communication i.e. message dispatching in...